Understanding Stack and Heap Memory in Zig
Learn the differences between stack and heap memory in Zig, and how to leverage them effectively for optimal performance and memory management.
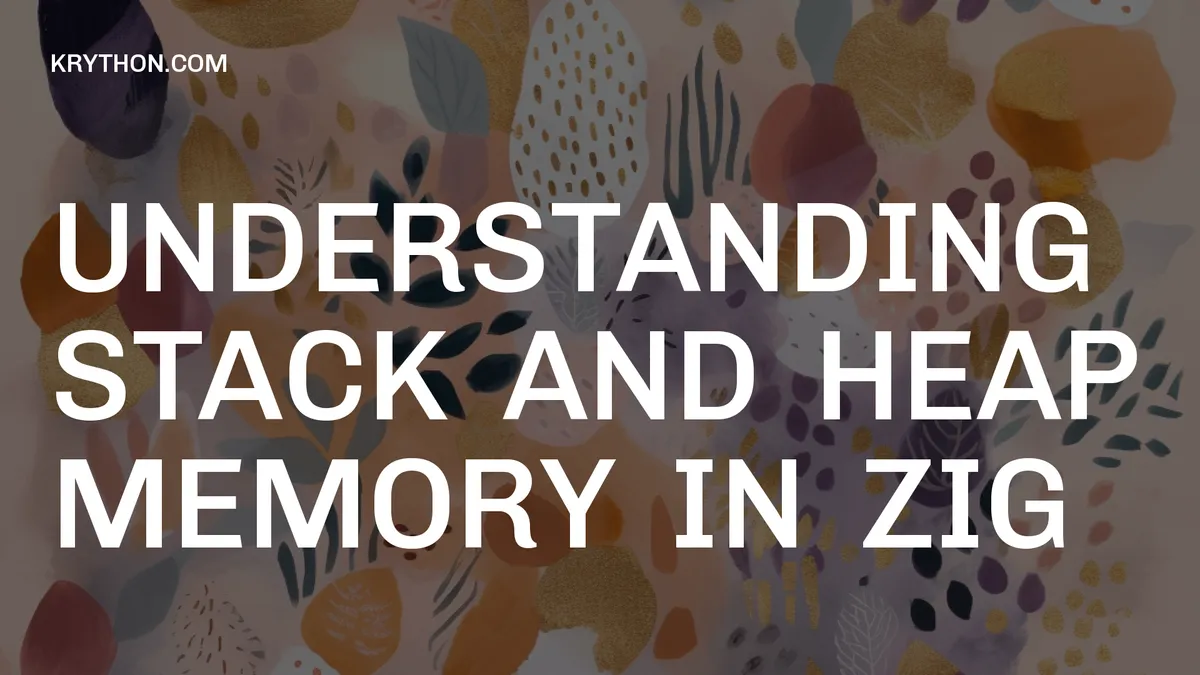
Introduction
In the world of programming languages, memory management is a crucial aspect that directly impacts the performance, efficiency, and reliability of your applications. Zig, a modern systems programming language, provides developers with explicit control over memory allocation, enabling them to choose between stack and heap memory based on their specific requirements.
Stack Memory
The stack is a region of memory that is automatically managed by the compiler and follows a last-in, first-out (LIFO) order. When a function is called, the local variables and function parameters are stored on the stack. Once the function completes its execution, the memory occupied by these variables is automatically deallocated, ensuring efficient memory usage.
In Zig, you can allocate memory on the stack by declaring variables with a fixed size. These variables are known as stack-allocated variables, and their lifetime is bound to the scope in which they are defined. Here's an examples:
- Struct Instances
const Point = struct {
x: f32,
y: f32,
};
pub fn main() void {
// Stack-allocated struct instance
var point: Point = .{
.x = 1.5,
.y = 2.7,
};
// Access and modify struct fields
point.x += 3.0;
point.y -= 1.2;
// 'point' is automatically deallocated when it goes out of scope
}
In this example, point
is a stack-allocated instance of the Point
struct. Its memory is automatically managed by the compiler, and it will be deallocated when the main
function returns.
- Fixed-size Arrays
pub fn main() void {
// Stack-allocated fixed-size array
var numbers: [5]i32 = .{ 10, 20, 30, 40, 50 };
// Access and modify array elements
numbers[2] = 999;
// 'numbers' is automatically deallocated when it goes out of scope
}
Here, numbers
is a stack-allocated fixed-size array of i32
values. Its size is known at compile-time, so it can be allocated on the stack.
Stack-allocated variables are excellent choices when you know the fixed size of the data at compile-time and when the lifetime of the data is limited to a specific scope. They provide efficient memory usage and predictable performance, making them suitable for scenarios where deterministic behavior is crucial.
Heap Memory
The heap is a region of memory that is dynamically allocated and deallocated during runtime. Unlike stack memory, which has a fixed size and is managed automatically, heap memory is more flexible and allows for dynamic allocation of varying memory sizes.
In Zig, you can allocate memory on the heap using the allocator
module, which provides functions for dynamic memory allocation and deallocation. Here's an examples:
- Dynamic Arrays
const std = @import("std");
pub fn main() !void {
const allocator = std.heap.page_allocator;
// Heap-allocated dynamic array using ArrayList
var numbers = std.ArrayList(u32).init(allocator);
defer numbers.deinit(); // This correctly frees the ArrayList and its elements
// Append elements to the array
try numbers.append(10);
try numbers.append(20);
try numbers.append(30);
// Use the array
// Since ArrayList does not implement the `any` type directly, we use `numbers.items` to get the slice
std.debug.print("Array: {any}\n", .{numbers.items});
// No need to manually free 'numbers', 'defer numbers.deinit()' handles it
}
In this example, we create a dynamic array of u32
values using heap memory. The allocator.alloc
function is used to allocate memory for the array, and the append
method is used to add elements dynamically. We need to manually free the memory using allocator.free
when we're done with the array.
It's important to note that when allocating memory on the heap, you are responsible for manually deallocating it when it's no longer needed.
Heap-allocated memory is useful when you need dynamic data structures, such as arrays or slices with variable sizes, or when the lifetime of the data extends beyond a single scope or function call. However, it comes with the overhead of dynamic memory allocation and deallocation, which can impact performance if not managed properly.
When to Use Stack vs. Heap Memory
Choosing between stack and heap memory is a trade-off between performance, flexibility, and memory usage. Here are some general guidelines:
Use Stack Memory When:
- You know the fixed size of the data at compile-time.
- The lifetime of the data is limited to a specific scope or function call.
- You need deterministic and predictable performance.
- You want to minimize dynamic memory allocation overhead.
Use Heap Memory When:
- You need dynamic data structures with variable sizes (e.g., dynamic arrays, linked lists).
- The lifetime of the data extends beyond a single scope or function call.
- You need to allocate and deallocate memory dynamically at runtime.
- You're willing to trade some performance for flexibility and dynamic memory management.
It's important to note that both stack and heap memory have their advantages and drawbacks. In some cases, you might need to use a combination of both to achieve the desired functionality and performance characteristics.
Conclusion
In Zig, understanding the differences between stack and heap memory is crucial for effective memory management and optimizing your applications. Stack memory offers efficient and predictable performance but is limited to fixed-size data with a well-defined lifetime. Heap memory provides dynamic memory allocation and flexibility but comes with the overhead of runtime memory management.
By carefully considering the requirements of your application and the trade-offs between performance, flexibility, and memory usage, you can make informed decisions about when to use stack or heap memory in your Zig programs. This knowledge will enable you to write efficient, reliable, and high-performance systems code.