Explaining Promise.reject in Node.js for Handling Errors
Explore practical applications in error handling, promise chaining, and asynchronous operations for robust, efficient code.
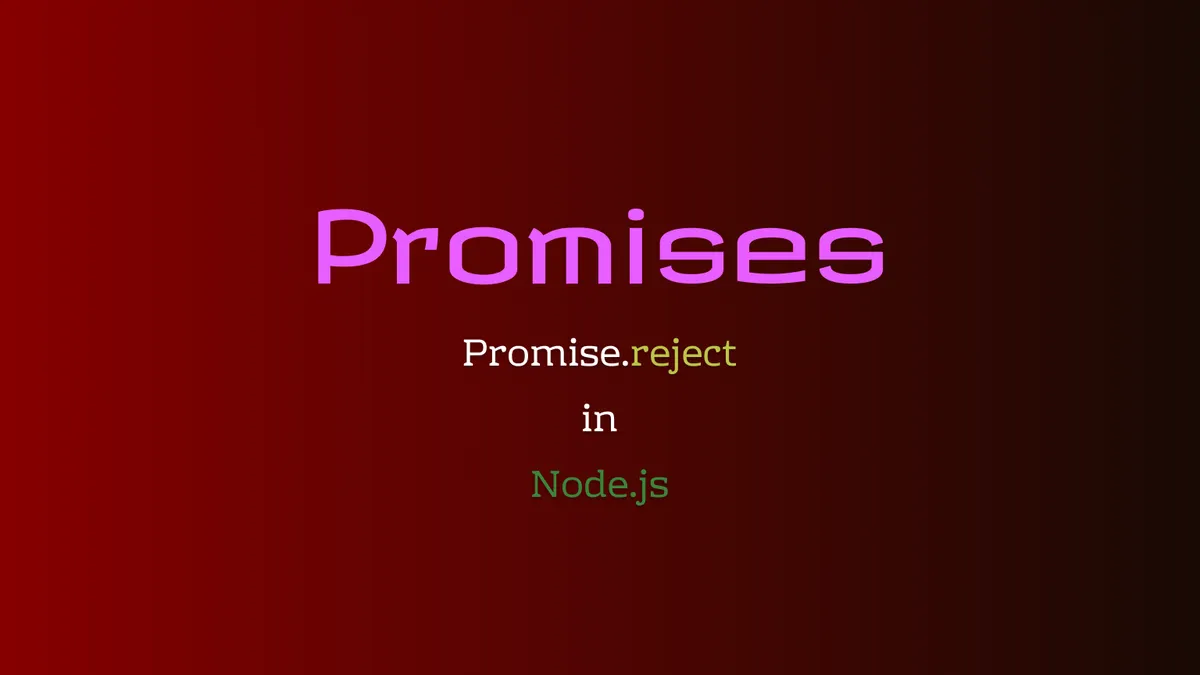
Introduction
Promises in JavaScript are a way to handle asynchronous operations in a more organized and readable manner. They are a way to handle the errors and results of an asynchronous operation in a single place. ThePromise.reject()
function is a way to create a promise that is already rejected, which can be useful in certain situations.
Step 1: Import the promise
module
The first step in using Promise.reject
in Node.js is to import the promise
module. This module is built-in to Node.js and does not need to be installed separately. You can import the module using the following code:
const Promise = require('promise');
Step 2: Create a rejected promise using Promise.reject()
To create a rejected promise, use the Promise.reject()
function and pass in the value that you want to be the reason for the rejection. For example:
const rejectedPromise = Promise.reject("Error message");
Step 3: Attach a rejection handler using .catch()
You can then attach a rejection handler to the promise using the .catch()
method, which takes a single argument—a function that will be called if the promise is rejected.
rejectedPromise.catch((error) => {
console.log(error);
});
Step 4: Attach a resolution handler using .then()
You can also use the.then()
method, which also takes a function as an argument, but this function is called if the promise is resolved.
rejectedPromise.then((result) => {
console.log("This will not be called");
});
Step 5: Examples of using Promise.reject
in a Node.js application
- Example 1: Handling user authentication
const authenticateUser = (username, password) => {
return new Promise((resolve, reject) => {
if(username !== 'admin' || password !== 'password') {
reject("Error: Invalid username or password");
} else {
resolve("Authentication successful");
}
});
}
authenticateUser("admin", "wrongpassword")
.then((message) => {
console.log(message);
})
.catch((error) => {
console.log(error);
});
In this example, the functionauthenticateUser()
returns a promise that checks if the provided username and password match the expected values. If they do not match, it uses Promise.reject()
to return a rejected promise with the error message "Error: Invalid username or password". The.catch()
method is then used to handle the rejection and display the error message.
- Example 2: Handling file upload
const uploadFile = (file) => {
return new Promise((resolve, reject) => {
if(file.size > 5000000) {
reject("Error: File size exceeds the maximum limit of 5MB");
} else {
resolve("File uploaded successfully");
}
});
}
const file = {
size: 6000000
}
uploadFile(file)
.then((message) => {
console.log(message);
})
.catch((error) => {
console.log(error);
});
In this example, the functionuploadFile()
returns a promise that checks if the file size is within the maximum limit of 5MB. If it exceeds the limit, it uses Promise.reject()
to return a rejected promise with the error message "Error: File size exceeds the maximum limit of 5MB". The.catch()
method is then used to handle the rejection and display the error message.
- Example 3: Handling API call
const makeApiCall = (url) => {
return new Promise((resolve, reject) => {
fetch(url)
.then(response => {
if(!response.ok) {
reject(`Error: ${response.statusText}`);
} else {
resolve(response.json());
}
})
.catch(error => {
reject(`Error: ${error}`);
});
});
}
makeApiCall("https://api.example.com/data")
.then((data) => {
console.log("Data:", data);
})
.catch((error) => {
console.log("Error:", error);
});
In this example, the functionmakeApiCall()
returns a promise that makes an API call to the provided URL using fetch()
. If the API call is not successful, it uses Promise.reject()
to return a rejected promise with the error message "Error: [status text]" or "Error: [error message]". The.catch()
method is then used to handle the rejection and display the error message.
Conclusion
The Promise.reject()
function in JavaScript is a powerful tool for handling errors in a more organized and readable manner. It allows developers to create rejected promises that can be handled using the .catch()
method, providing a clear way to handle errors in asynchronous operations. The examples provided in this article demonstrate how Promise.reject()
can be used in different scenarios, such as user authentication, file uploads, and API calls. When using Promise.reject()
it's important to make sure to have a catch block that can handle the rejected promise and provide appropriate feedback to the user. By following the guidelines and best practices outlined in this article, developers can effectively use the Promise.reject()
function to improve error handling in their code.