Using Promise.resolve in Node.js: Simple Walkthrough
Explore Promise.resolve in JavaScript. Manage non-blocking tasks effectively for browser or Node.js environments.
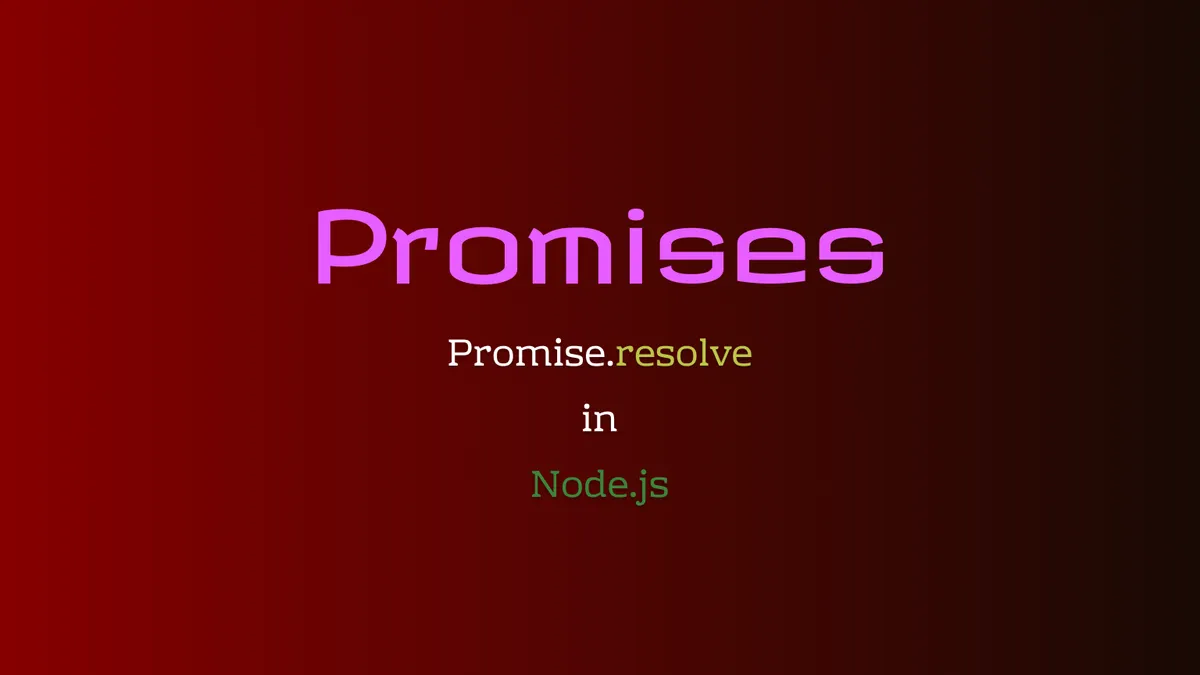
Introduction
Promise.resolve
is a method in JavaScript that is used to create a new promise that is resolved with a given value. This method is part of the JavaScript Promise API and is often used in Node.js applications to handle asynchronous operations. In this article, we will explore how to use Promise.resolve
in Node.js and provide examples to illustrate its usage.
Step 1: Import the promise
module
The first step in using Promise.resolve
in Node.js is to import the 'promise' module. This module is built-in to Node.js and does not need to be installed separately. You can import the module using the following code:
const Promise = require('promise');
Step 2: Use the Promise.resolve
method
Once you have imported the promise module, you can use the Promise.resolve
method to create a new promise that is resolved with a given value. The basic syntax of the method is as follows:
Promise.resolve(value);
value
is the value that the promise should be resolved with.
- Example 1:
const myPromise = Promise.resolve('Hello, World!');
In this example, we create a new promise called myPromise
that is resolved with the value Hello, World!.
- Example 2:
const myPromise = Promise.resolve(new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Hello, World!');
}, 1000);
}));
In this example, we create a new promise called myPromise
that is resolved with the value of another promise. In this case, the inner promise is resolved with Hello, World! after 1 second.
Step 3: Consume the promise
Once you have created a promise using the Promise.resolve
method, you can consume the promise by chaining a .then
method to it. The .then
method allows you to specify a callback function that will be called when the promise is resolved.
- Example 1:
myPromise.then((value) => {
console.log(value);
});
In this example, the callback function passed to the .then
method logs the resolved value of the promise to the console.
- Example 2:
myPromise.then((value) => {
return value + ' from Node.js!';
}).then((value) => {
console.log(value);
});
In this example, we chain two .then
methods together. The first .then
method takes the resolved value of the promise and appends the string from Node.js! to it. The second .then
method logs the modified value to the console.
Step 4: Examples of using Promise.resolve
in a Node.js application
- Example 1: Reading a file asynchronously
const Promise = require('promise');
const fs = require('fs');
const myPromise = Promise.resolve(new Promise((resolve, reject) => {
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) {
reject(err);
} else {
resolve(data);
}
});
}));
myPromise.then((data) => {
console.log(data);
}).catch((err) => {
console.log(err);
});
This is a NodeJS
code
In this example, we use the fs
module to read the contents of a file asynchronously. We create a new promise using the Promise.resolve
method that is resolved with the contents of the file. The .then
method is used to log the contents of the file to the console and the .catch
method is used to catch any errors that might occur during the file reading process.
- Example 2: Making an HTTP request
const Promise = require('promise');
const https = require('https');
const myPromise = Promise.resolve(new Promise((resolve, reject) => {
https.get('https://jsonplaceholder.typicode.com/posts', (res) => {
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
resolve(JSON.parse(data));
});
}).on('error', (err) => {
reject(err);
});
}));
myPromise.then((data) => {
console.log(data);
}).catch((err) => {
console.log(err);
});
In this example, we use the https
module to make an HTTP request to a JSON placeholder API. We create a new promise using the Promise.resolve
method that is resolved with the JSON data returned from the API. The .then
method is used to log the JSON data to the console and the .catch
method is used to catch any errors that might occur during the HTTP request.
- Example 3: Handling a database query
const Promise = require('promise');
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'test'
});
const myPromise = Promise.resolve(new Promise((resolve, reject) => {
connection.query('SELECT * FROM users', (err, result) => {
if (err) {
reject(err);
} else {
resolve(result);
}
});
}));
myPromise.then((data) => {
console.log(data);
}).catch((err) => {
console.log(err);
});
connection.end();
This is a NodeJS
code
In this example, we use the mysql
module to query a MySQL database. We create a new promise using the Promise.resolve
method that is resolved with the result of the query. The .then
method is used to log the query result to the console and the .catch
method is used to catch any errors that might occur during the database query. Additionally, after the promise is handled, we close the connection to the database using the connection.end()
method.
Conclusion
the Promise.resolve
method in Node.js is a powerful tool for handling asynchronous operations. It allows you to create a new promise that is resolved with a given value, making it easy to specify the behavior of your code when the promise is fulfilled. We have discussed how to import the promise module, use the Promise.resolve
method, and consume the promise, with real-world examples to illustrate its usage. Additionally, we have provided some tips and best practices to help you get the most out of using Promise.resolve
in your Node.js applications. By following the guidelines and examples outlined in this article, you should be well-equipped to start using Promise.resolve
in your own projects and effectively handle asynchronous operations.