Manipulating files and directories with fs-extra in Node.js
Learn how to use the fs-extra library in Node.js to manipulate files and directories in an efficient and flexible way.
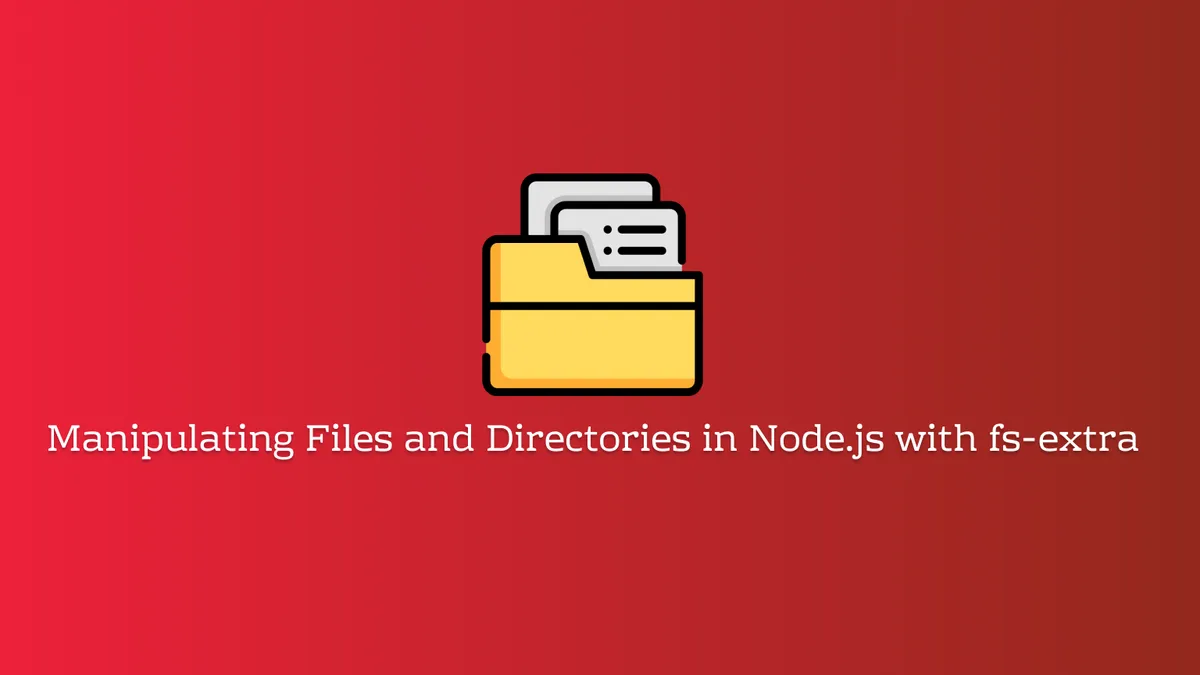
Introduction
fs-extra
is a third-party library for Node.js that builds on the functionality provided by the built-in fs
(file system) module. It provides a set of additional methods for working with the file system that are not included in the built-in module. These include methods for copying, moving, and removing files and directories, as well as methods for creating and modifying the properties of files and directories. It also provides async versions of the same functions for non-blocking operation.
With fs-extra
, you can work with the file system in a more efficient and flexible way. It provides a set of high-level abstractions for common file system operations, so you don't have to write low-level code. It also makes error handling simpler by catching and handling errors at the appropriate level of abstraction. You will have more powerful features than the core fs module such as handling errors in a better way, ability to work with async methods and also it provides more than the core fs methods.
Step 1: Installing fs-extra
To use fs-extra
in your Node.js project, you will first need to install it using npm
. Open your terminal, navigate to your project's root directory, and run the following command:
npm install --save fs-extra
This will install fs-extra
and add it to your project's dependencies.
Step 2: Importing fs-extra
Once you have installed fs-extra
, you can import it in your Node.js code by using the require
function. For example:
const fs = require('fs-extra');
Step 3: Copying files and directories
One of the most common tasks when working with the file system is copying files and directories. fs-extra
provides a copy method that makes it easy to copy files and directories. For example, to copy
a file from one location to another, you can use the following code:
fs.copy('/path/to/source/file.txt', '/path/to/destination/file.txt', (err) => {
if (err) return console.error(err);
console.log("file was copied");
});
You can also copy a directory and all its contents by passing the directory path to the copy
method. For example:
fs.copy('/path/to/source/directory', '/path/to/destination/directory', (err) => {
if (err) return console.error(err);
console.log("directory was copied");
});
Step 4: Removing files and directories
Another common task when working with the file system is removing files and directories. fs-extra
provides a remove
method that makes it easy to remove files and directories. For example, to remove a file, you can use the following code:
fs.remove('/path/to/file.txt', (err) => {
if (err) return console.error(err);
console.log("file was removed");
});
You can also remove a directory and all its contents by passing the directory path to the remove
method. For example:
fs.remove('/path/to/directory', (err) => {
if (err) return console.error(err);
console.log("directory was removed");
});
Step 5: Renaming files and directories
fs-extra
also provides a rename
method that makes it easy to rename files and directories for example, to rename a file, you can use the following code:
fs.rename('/path/to/oldFile.txt', '/path/to/newFile.txt', (err) => {
if (err) return console.error(err);
console.log("file was renamed");
});
You can also rename a directory by passing the current and new directory path to the rename
method.
Step 6: Creating directories
fs-extra
also provides a mkdir
method that can be used to create a new directory. This method can also create multiple nested directories in one call. For example:
fs.mkdir('/path/to/directory', { recursive: true }, (err) => {
if (err) return console.error(err);
console.log("directory was created");
});
This method also accepts a second argument, which is an options object. By setting the recursive
option to true, it will create all the nested directories in the provided path.
Step 7: Reading and Writing files
fs-extra
also provides methods for reading and writing files, such as readFile
, writeFile
, and appendFile
. These methods can be used to read and write both text and binary files. For example, to read a text file:
fs.readFile('/path/to/file.txt', 'utf-8', (err, data) => {
if (err) return console.error(err);
console.log(data);
});
And to write a text file:
fs.writeFile('/path/to/file.txt', 'Hello World!', (err) => {
if (err) return console.error(err);
console.log("file was written");
});
And to append data to an existing file:
fs.appendFile('/path/to/file.txt', '\nThis is a new line.', (err) => {
if (err) return console.error(err);
console.log("data was appended to the file");
});
This method will add new data to the end of the file without overwriting the existing data.
Step 8: Closing file descriptor
When you open a file, the operating system assigns a file descriptor to the file, which is an integer that represents the open file. It is important to close the file descriptor after you are done with the file to free up system resources.
fs-extra
provides the close
method that can be used to close a file descriptor. This method takes two arguments, the file descriptor and a callback function that will be called when the file descriptor is closed.
For example, to close a file descriptor after reading a file:
fs.open('/path/to/file.txt', 'r', (err, fd) => {
if (err) return console.error(err);
// read file here
fs.close(fd, (err) => {
if (err) return console.error(err);
console.log("file descriptor was closed");
});
});
It is also worth noting that in most cases the file descriptor is closed automatically when the file is closed, but it is good practice to close the descriptor explicitly after use.
Step 9: Handling errors
It is important to handle errors properly when working with the file system, as unexpected errors can cause your application to crash or result in data loss. fs-extra
methods typically take a callback function as their last argument, which is called when the operation is complete or an error occurs.
For example, when reading a file, it's important to check for errors and handle them accordingly:
fs.readFile('/path/to/file.txt', 'utf-8', (err, data) => {
if (err) {
if (err.code === 'ENOENT') {
console.error('File not found.');
} else {
console.error(err);
}
return;
}
console.log(data);
});
In this example, if an error occurs when reading the file, the callback function will check the error code and provide a more specific message for the "File not found" error. For other errors, it will print the error message to the console.
It's also a good practice to always include an error handling mechanism in every step of your code when working with files. This will make it easier to identify and fix errors, and prevent data loss or unexpected behavior in your application.
Conclusion
fs-extra
is a great module for working with files and directories in Node.js. It provides a wide range of functionality that is not available in the core fs
module, and it makes it much easier to work with files and directories in your Node.js applications. By using fs-extra
, you can copy, remove, rename, create, read and write files and directories easily and efficiently. It is a great tool to have in your Node.js toolbox and can help you write more robust and maintainable code.