Node.js File System: Understanding and Manipulating Files and Directories
Explore the capabilities of the Node.js file system module and learn how to use it.
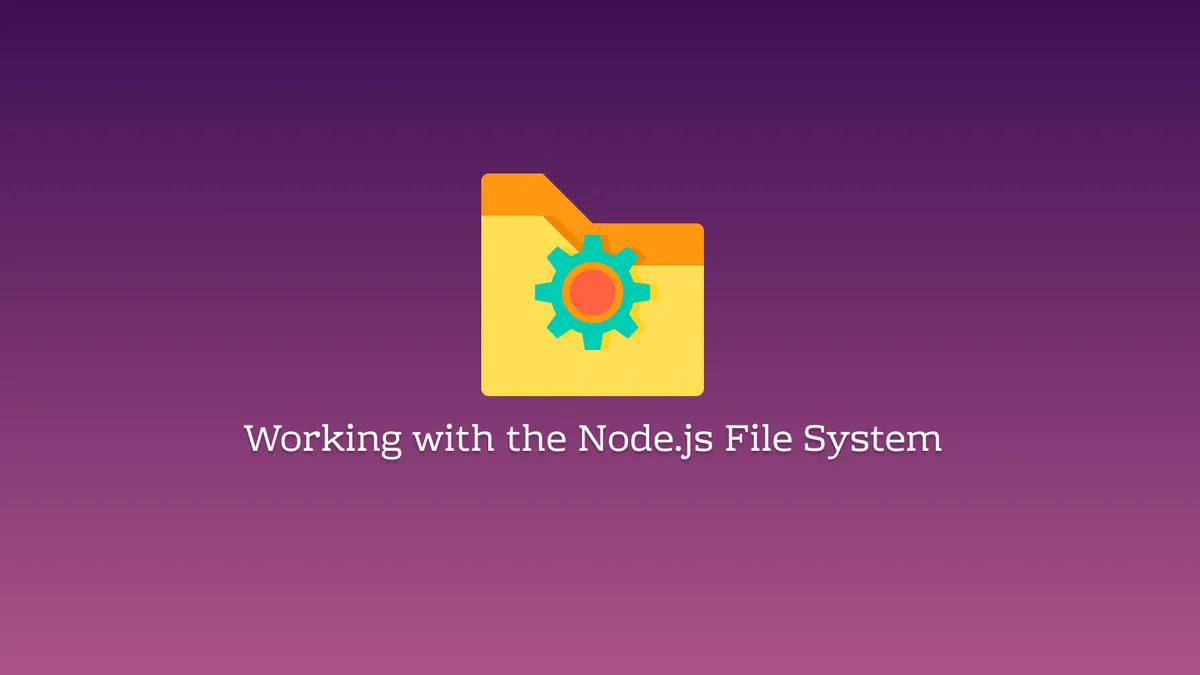
Introduction
The Node.js file system module, fs, provides a way to work with the file system on a computer. This module allows developers to read, write, and manipulate files and directories in a Node.js application. In this article, we'll take a look at some of the methods provided by the fs module, as well as tips for working with the file system in a Node.js application.
Step 1: Import the File System module
Before you can start working with the file system in Node.js, you need to import the fs
module. You can do this by adding the following line at the top of your JavaScript file:
const fs = require('fs');
Step 2: Reading a file
Once you have the fs
module imported, you can use it to read files. The fs.readFile()
method is used to read files in Node.js. It takes two arguments: the file path and a callback function. The callback function is passed two arguments: an error object and the contents of the file. Here's an example of how to use fs.readFile()
:
fs.readFile('file.txt', (err, data) => {
if (err) {
throw err;
};
console.log(data.toString());
});
In this example, we're reading the file "file.txt" and using the callback function to log the contents of the file to the console.
Step 3: Writing to a file
To write to a file in Node.js, you can use the fs.writeFile()
method. This method takes three arguments: the file path, the data to write, and a callback function. If the file doesn't exist, it will be created, and if it does exist, its contents will be overwritten. Here's an example of how to use fs.writeFile()
:
fs.writeFile('file.txt', 'Hello World!', (err) => {
if (err) throw err;
console.log('The file has been saved!');
});
In this example, we're writing the string Hello World!
to the file file.txt
and using the callback function to log a message to the console once the file has been saved.
Step 4: Appending data to a file
In addition to reading and writing files, the fs module also allows you to append data to an existing file. To append data to a file, you can use the fs.appendFile()
method. This method takes three arguments: the file path, the data to append, and a callback function. If the file doesn't exist, it will be created, and if it does exist, the data will be appended to the end of the file. Here's an example of how to use fs.appendFile()
:
fs.appendFile('file.txt', 'Appended Data', (err) => {
if (err) throw err;
console.log('Data appended to file!');
});
In this example, we're appending the string Appended Data
to the file file.txt
and using the callback function to log a message to the console once the data has been appended.
You can also use the fs.open()
method along with the fs.write()
method for appending data. First, open the file with the fs.open()
method and pass in the file path and the flag 'a' (for appending). This will return a file descriptor which you can use to write to the file using the fs.write()
method.
fs.open('file.txt', 'a', (err, fd) => {
if (err) throw err;
fs.write(fd, ' Appended Data', (err) => {
if (err) throw err;
fs.close(fd, (err) => {
if (err) throw err;
console.log('Data appended and file descriptor closed');
});
});
});
This will open the file in append mode, then writes the data and closes the file descriptor after data has been written.
It's worth noting that both these methods are synchronous, so if you are going to work with large files, or many files, it is recommended to use third party libraries like fs-extra
, graceful-fs
etc, that provide async versions of the same functions or even provide more powerful features.
Keep in mind that in order to append data to a file, you must have write permission for the file, if you do not have permission to write to file, the method will throw an error, thus it's important to handle those errors and take appropriate action.
Step 5: Manipulating files and directories
In addition to reading and writing files, the fs module also provides methods for manipulating files and directories. For example, the fs.rename()
method can be used to rename a file, and the fs.unlink()
method can be used to delete a file. Similarly, fs.mkdir()
can be used to create a directory and fs.rmdir()
can be used to remove a directory.
Example 1: Renaming a file using fs.rename()
The fs.rename()
method can be used to rename a file. It takes two arguments: the current file name and the new file name. Here's an example of how to use fs.rename()
to rename a file named oldFileName.txt
to newFileName.txt
:
fs.rename('oldFileName.txt', 'newFileName.txt', (err) => {
if (err) throw err;
console.log('File Renamed!');
});
This method is synchronous and can throw an error in case the file with the old name doesn't exist, or if the new file name is already being used by another file, or you do not have permission to rename the file, thus it's important to handle those errors and take appropriate action.
Example 2: Deleting a file using fs.unlink()
The fs.unlink()
method can be used to delete a file. It takes the file path as an argument. Here's an example of how to use fs.unlink()
to delete a file named fileToDelete.txt
:
fs.unlink('fileToDelete.txt', (err) => {
if (err) throw err;
console.log('File deleted!');
});
This method is synchronous and can throw an error if the file doesn't exist or if you do not have permission to delete the file, thus it's important to handle those errors and take appropriate action.
Example 3: Creating a directory using fs.mkdir()
The fs.mkdir()
method can be used to create a directory. It takes the directory path and an optional options object as arguments. Here's an example of how to use fs.mkdir()
to create a directory named newDirectory
:
fs.mkdir('newDirectory', { recursive: true }, (err) => {
if (err) throw err;
console.log('Directory created!');
});
In this example, we're passing the recursive option as true, which means that the method will create any necessary parent directories before creating the new directory. If the directory already exists, this method will throw an error, thus it's important to handle those errors and take appropriate action.
Example 4: Removing a directory using fs.rmdir()
The fs.rmdir()
method can be used to remove a directory. It takes the directory path as an argument. Here's an example of how to use fs.rmdir()
to remove a directory named oldDirectory
:
fs.rmdir('oldDirectory', (err) => {
if (err) throw err;
console.log('Directory removed!');
});
This method is synchronous and can throw an error if the directory doesn't exist, it's not empty or you do not have permission to delete the directory, thus it's important to handle those errors and take appropriate action.
Step 6: Closing file descriptor
It's a good practice to close the file descriptor after you are done reading or writing the file, which can be done using the fs.close()
method. It not only frees up resources but also allows other processes to access the same file descriptor. Here's an example of how to use fs.close()
to close a file descriptor:
fs.open('file.txt', 'r', (err, fd) => {
if (err) throw err;
// Work with file
fs.close(fd, (err) => {
if (err) throw err;
console.log('File descriptor closed.');
});
});
In this example, we're opening the file file.txt
in read mode and getting a file descriptor. Then, after we've finished working with the file, we're closing the file descriptor. It's a best practice to always close the file descriptor after you're done using it to ensure that resources are freed up properly.
Step 7: Handling errors
It's important to handle errors that may occur when working with the file system. For example, trying to read a file that doesn't exist or doesn't have the proper permissions will result in an error. Using the callback functions provided by the fs module's methods can help with this by allowing you to catch and handle errors that occur during file system operations.
Here is an example of how to handle error when trying to read a file
fs.readFile('file.txt', (err, data) => {
if (err) {
console.error(`An error occurred: ${err}`);
return;
}
console.log(data.toString());
});
In this example, we're using the callback function passed to fs.readFile()
to check for an error. If an error occurred, we're logging an error message to the console and returning.
Step 8: Using third-party libraries
In addition to the core fs module, there are also several third-party libraries available that provide additional functionality for working with the file system in Node.js. Some of these libraries, like fs-extra
and graceful-fs
, provide additional methods for tasks like copying and moving files, as well as making it easier to work with async and non-blocking operations.
Conclusion
The Node.js file system module provides a powerful set of tools for working with files and directories in a Node.js application. With the variety of methods provided by the fs module, you can easily read, write and manipulate files and directories. Additionally, handling errors and closing file descriptor, keeping non-blocking operations in mind by using third party libraries are best practices to keep in mind while working with Node.js file system. By mastering the Node.js file system module and understanding how to use it effectively, you'll be well equipped to handle a wide range of file system tasks in your Node.js applications.